Token overview
This guide provides an overview of the authentication/authorization process, explains the two types of tokens we leverage with our products, and covers some best practices.
Authentication & authorization
For you to connect your end-user’s data to your app, the end-user must authenticate with their data provider (bank or financial institution) and authorize data to be shared. Initial authentication remains in effect until either the end-user revokes access to their data or their associated refresh token expires. Akoya uses an OAuth 2.0 flow with OpenID Connect (OIDC) authentication protocol in the following steps:
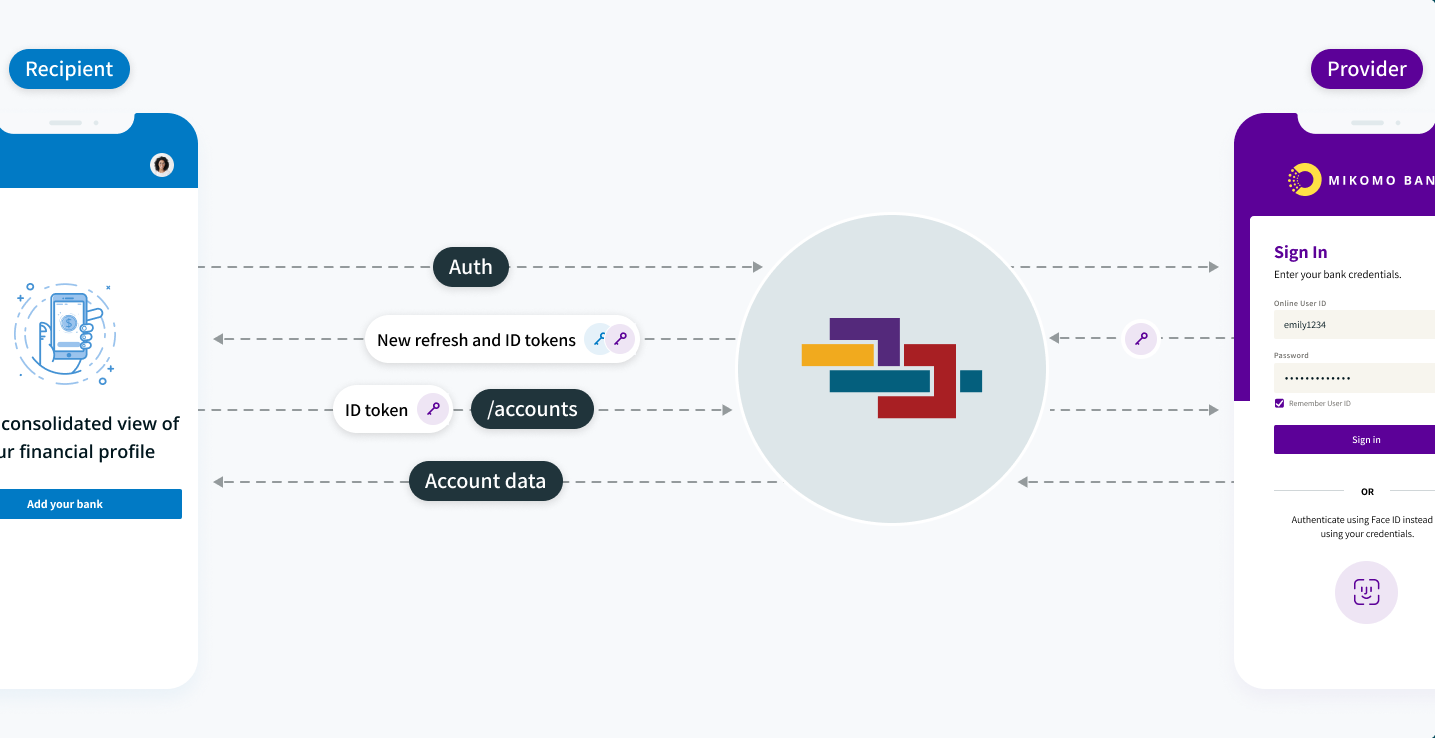
Token flow
Authorization Endpoint
Your app sends the end-user to their provider’s login page via Akoya using a specific URL with required parameters for authentication. On success, an authorization code is granted to your app.
Start by sending the user to the authorization endpoint:
GET https://sandbox-idp.ddp.akoya.com/auth
?connector={Data Provider identifier provided by Akoya} // - Required
&client_id={client ID} // - Required, provided in the Data Recipient Hub
&redirect_uri={Redirect URI} // - Required, set by you in the Data Recipient Hub
&response_type=code // - Required
&scope=openid email profile offline_access // - Required
&state={unique, randomly generated, opaque, and non-guessable string} // - Required
Find more details in Get authorization code.
The end-user completes the authorization consent with their provider. At this point, Akoya sends the end-user to your app’s redirect_uri
with the authorization code and state. In the event an error occurs, or the user declines consent, please review error scenarios here.
HTTP/1.1 302 Found
Location: {Your redirect URI as specified in the authorization endpoint}
?code={Authorization Code} // - Code valid for 5 minutes to be exchanged for token
&state={Arbitrary String} // - String matching the state provided in the authorization endpoint
To prevent possible CSRF attacks you should validate your state param. You can find more information about this important security step here: https://auth0.com/docs/secure/attack-protection/state-parameters
Example URL sent to your app with authorization code:
https://recipient.ddp.akoya.com/flow/callback?code=vhmji7kmopeil4jyb57wc4znx&state=
Authorization Code for Token Exchange
With the authorization code and your app’s details, use the Token endpoint to retrieve a set of tokens (ID token and refresh token). These tokens allow you permissioned access to the end-user’s data.
POST https://sandbox-idp.ddp.akoya.com/token
Content-Type: application/x-www-form-urlencoded
grant_type=authorization_code // - Required
&code={Authorization Code} // - Required
&redirect_uri={Redirect URI} // - Required, must match the one in the authorization endpoint
Success Response:
HTTP/1.1 200 OK
Content-Type: application/json;charset=UTF-8
{
"token_type": "bearer",
"expires_in": {Lifetime In Seconds},
"refresh_token": "{Refresh Token}",
"id_token": "{ID Token}"
}
Should an error occur, please consult the token error documentation here.
ID Token
Akoya will issue your app an ID token (OIDC token—a signed JSON Web Token). The ID Token is a short-term token used for requesting data from Akoya product endpoints.
This means the ID token will function as your access (bearer) token. The /token
endpoint will not return an explicit field called access_token
. This is called out as some libraries and tools may need adjustments or won't handle this correctly out of the box. For example, Postman currently expects there to be an explicit field access_token
field for the built in OAuth2.0 generic handler. In the Akoya postman collection, this will be handled for you. Once the token is created using the environment file and test code, the id_token
is set to be used in all the requests as the bearer token for the authorization header.
Tokens are granted for each end-user for your specific app and are specific to their current account authorization from their provider. Please see the unique keys and values guide for specifics as to the level of specificity of the token and elements. This token may be decoded to view identity token claims (see ID token details) which may be helpful in more advanced usage scenarios.
The ID token lifetime may last up to 24 hours. However, providers often have a shorter expiration for ID tokens.
Note
We recommend treating ID tokens as having a life of no longer than 15 minutes and coding to automatically refresh if the ID token has expired.
Make a refresh token call to renew the tokens if they expire or after an app uses an ID token for 15 minutes to follow this recommendation.
Refresh Token
The refresh token is a longer-term token and is tied to the end-user’s authorization. Refreshing tokens allows your app to replace an expired ID token without asking the end-user to reauthenticate and maintains authorization when the end-user is not actively using your app. The refresh token is not a JWT token and thus, cannot be decoded.
Refresh tokens are used with the Token API and not as bearer tokens in Akoya product calls. A call to the Token API to refresh tokens issues a new ID token and the existing refresh token.
The refresh token must be treated as highly confidential
Treat the refresh token as sensitive information. Each end-user token should be kept secure by using encrypted credentials or server-side storage.
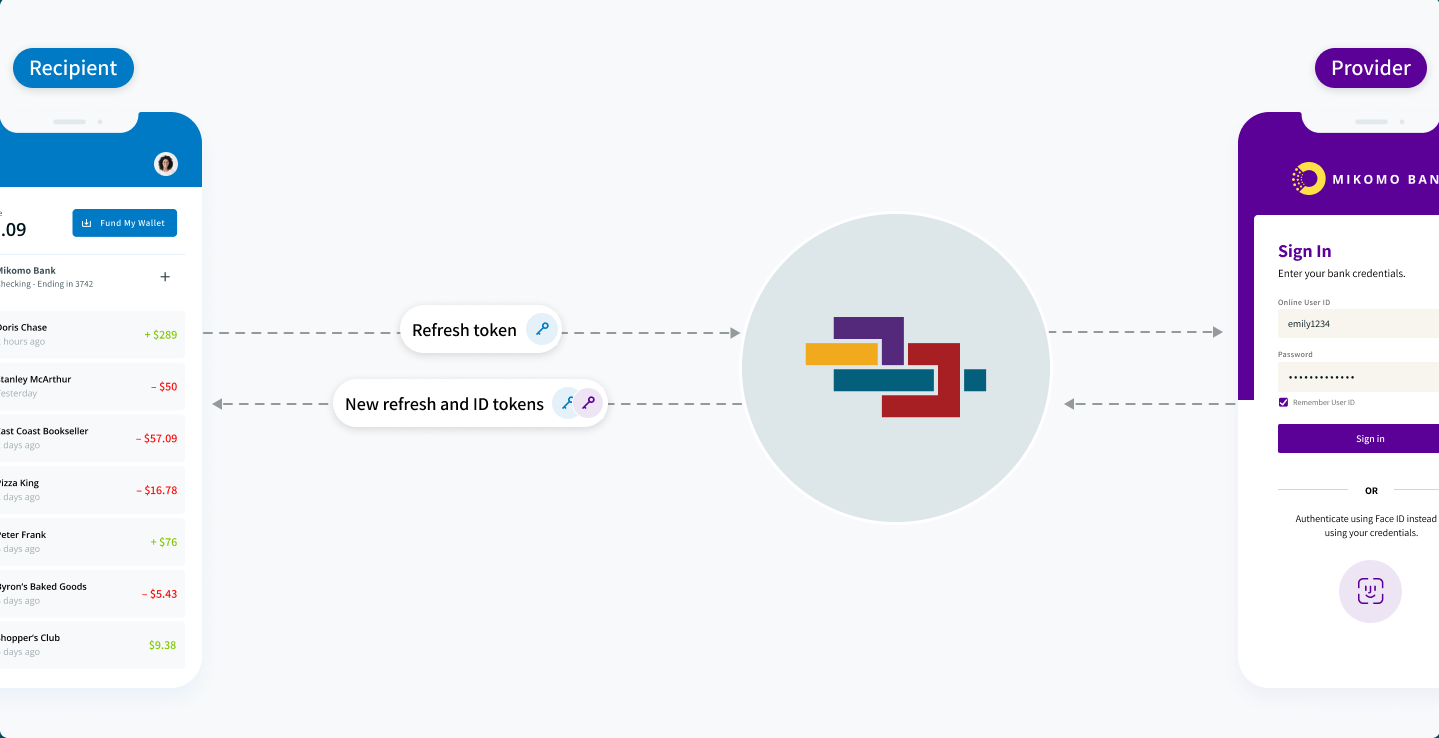
Refresh token calls return new refresh and ID tokens
Refresh token lifetime
Refresh tokens may be perpetual, have a rolling expiration, or have a set expiration from the date of authorization. Specific provider token expiration times are documented in the Data Recipient Hub, however the Akoya UX best practice is to code to proper error handling as tokens may expire earlier than the stated expiration times.
- Perpetual. The provider has no set expiration date for the refresh token.
- Set expiration. Some tokens have a set expiration period that forces reauthentication after the time indicated. This is commonly a year. After the token’s expiration, the end-user will need to go through the consent flow again regardless of any activity using the token.
- A rolling expiration. The token duration is reset every time the token is used. For instance, if your end-user’s bank has specified a rolling 6-month token expiration, you can refresh tokens without reauthentication at any time before the six-month expiration. On refresh, the token expiration will reset to six months from that time. However, if your app hasn’t refreshed tokens in 6 months, the next time the end-user uses your app, they will need to go through the consent flow again.
Token usage best practice
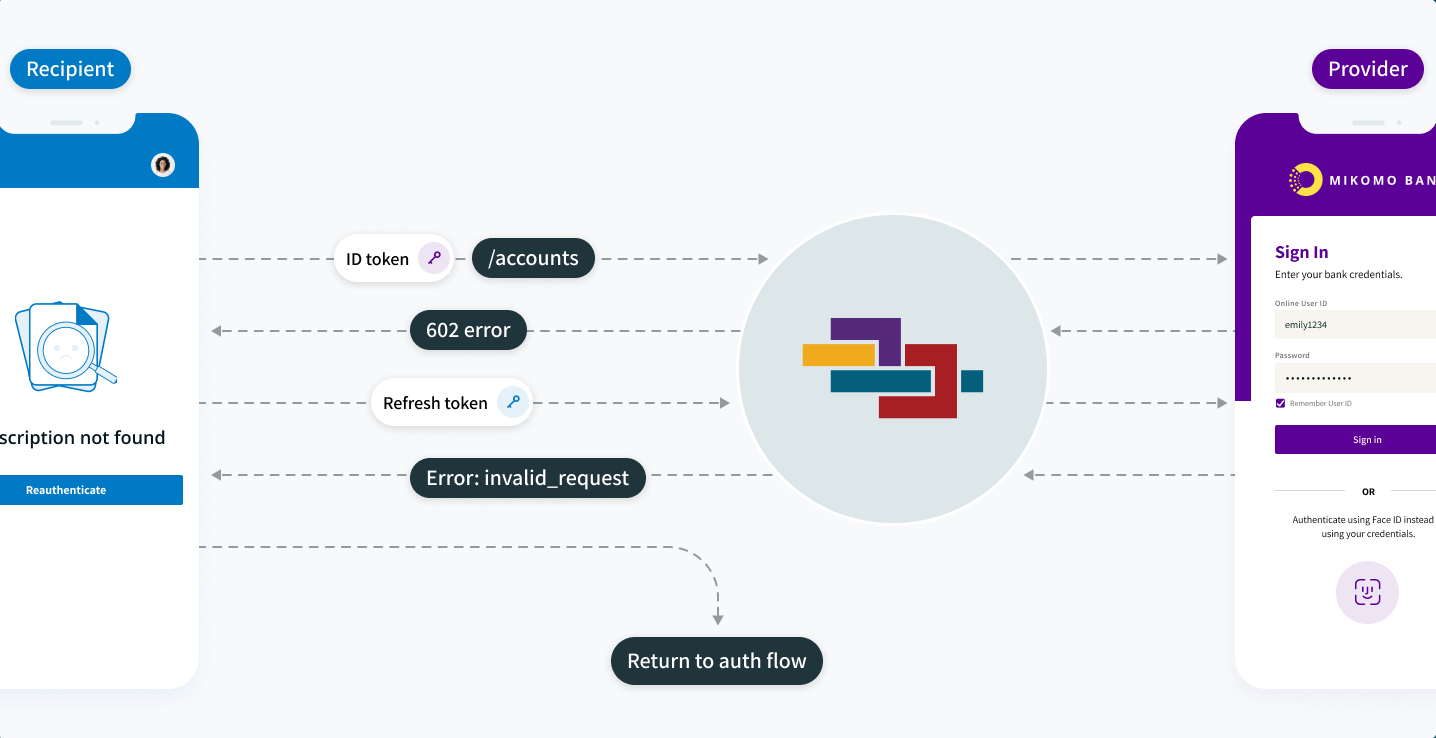
A possible token flow if both ID and refresh tokens are expired
The above diagram shows the two levels where tokens can fail and how to proceed. The first level is failure during a data request, in this case /accounts
. The second level is when trying to refresh the ID token (the refresh token has expired).
Note
Akoya highly recommends using a wrapper class around your data request calls that will check the response for the expired token errors. This is will allow you to intercept the error before hitting application specific code preventing duplicate logic and allows you to handle either the refresh or reauthenticate flow, then continue where you left off.
Expired ID Token
If an app requests data through an Akoya product endpoint (such as Accounts Info) using an expired ID token, the app will receive a 602
error. During this scenario, the token may or may not be past the expiration time. It may even have just been used; however, an event occurred where the ID token is no longer valid. Any data request with an expired token will result in the follow error:
{
"code": 602,
"message": "Customer not authorized"
}
To best handle this, Akoya recommends using a generic wrapper around all data calls that specifically looks for the 602
. Upon receiving this error, the app should send a refresh token request to the Token API and then try the data request again with the updated ID Token returning the data to the initial data call.
Expired refresh token
If you make a request using the Token API with an expired refresh token, you will receive an invalid_request
error.
{
"error": "invalid_request",
"error_description": "Refresh token is invalid or has already been claimed by another client."
}
An expired refresh token requires the app to redirect the end-user back through the consent flow and account selection process to reauthorize and receive a new set of ID and refresh tokens.
Note
The best practice here is to prompt the user letting them know the connection has expired and they’ll need to reauthenticate to continue using your service.
This helps the user understand they are going to need to go through the consent flow. Upon completion, you can present a call-to-action to resume the previous activity. This approach helps you because you don’t need to pause the current state. You just need an indicator of where to direct the user once done. This is common industry behavior that your users should be accustomed to.
Stop the never ending refresh loop
Once you receive an invalid request error indicating the refresh token is no longer valid, we recommended you flag the link as broken and stop attempting to refresh. This will prevent unnecessary noise in your error logs if you are running batch processes.
When a token is deemed broken, the only way to correct it is to have the user go through the consent flow again.
Additional information on tokens
More information on consent flow/token standards
To support authorization and ensure data privacy, Akoya uses tokens to verify end-user identity by leveraging the following standards:
- OAuth 2.0. Protocol that controls authorization to access a protected resource like a web app or API service.
- OpenID Connect (OIDC). Layer used over OAuth 2.0 that helps authenticate users and convey information about them.
- JSON Web Tokens (JWT). Standardized container format used to securely transfer data for authentication and authorization.
Change log
Date | Overview |
---|---|
2024-Sept-04 | Modified the "Stop the never ending refresh loop" section. |
2024-Sept-03 | Added intro. |
2024-Jul-26 | Original |
Need help?
Check out our Developer Community, or visit the Support Center in the Data Recipient Hub.
Looking for provider nuance documentation?
All provider nuance documentation is available in the Data providers section in the Data Recipient Hub.
Still stuck?
For all production issues, submit a support ticket through the Data Recipient Hub. Our support team is standing by 24/7. Questions and non-production issues will be answered during business hours.
Updated 6 months ago